java.lang.Object
javafx.scene.Node
javafx.scene.Parent
javafx.scene.layout.Region
javafx.scene.control.Control
javafx.scene.control.ScrollPane
- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
A Control that provides a scrolled, clipped viewport of its contents. It
allows the user to scroll the content around either directly (panning) or
by using scroll bars. The ScrollPane allows specification of the scroll
bar policy, which determines when scroll bars are displayed: always, never,
or only when they are needed. The scroll bar policy can be specified
independently for the horizontal and vertical scroll bars.
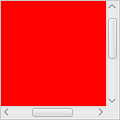
The ScrollPane allows the application to set the current, minimum, and
maximum values for positioning the contents in the horizontal and
vertical directions. These values are mapped proportionally onto the
layoutBounds
of the contained node.
ScrollPane layout calculations are based on the layoutBounds rather than the boundsInParent (visual bounds) of the scroll node. If an application wants the scrolling to be based on the visual bounds of the node (for scaled content etc.), it needs to wrap the scroll node in a Group.
ScrollPane sets focusTraversable to false.
This example creates a ScrollPane, which contains a Rectangle:
Rectangle rect = new Rectangle(200, 200, Color.RED);
ScrollPane s1 = new ScrollPane();
s1.setPrefSize(120, 120);
s1.setContent(rect);
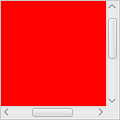
- Since:
- JavaFX 2.0
-
Property Summary
PropertiesTypePropertyDescriptionfinal ObjectProperty
<Node> The node used as the content of this ScrollPane.final BooleanProperty
If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport.final BooleanProperty
If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport.Specifies the policy for showing the horizontal scroll bar.final DoubleProperty
The maximum allowablehvalue
for this ScrollPane.final DoubleProperty
The minimum allowablehvalue
for this ScrollPane.final DoubleProperty
The current horizontal scroll position of the ScrollPane.final DoubleProperty
Specify the minimum height of the ScrollPane Viewport.final DoubleProperty
Specify the minimum width of the ScrollPane Viewport.final BooleanProperty
Specifies whether the user should be able to pan the viewport by using the mouse.final DoubleProperty
Specify the preferred height of the ScrollPane Viewport.final DoubleProperty
Specify the preferred width of the ScrollPane Viewport.Specifies the policy for showing the vertical scroll bar.final ObjectProperty
<Bounds> The actual Bounds of the ScrollPane Viewport.final DoubleProperty
The maximum allowablevvalue
for this ScrollPane.final DoubleProperty
The minimum allowablevvalue
for this ScrollPane.final DoubleProperty
The current vertical scroll position of the ScrollPane.Properties declared in class javafx.scene.control.Control
contextMenu, skin, tooltip
Properties declared in class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties declared in class javafx.scene.Parent
needsLayout
Properties declared in class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, focusVisible, focusWithin, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
An enumeration denoting the policy to be used by a scrollable Control in deciding whether to show a scroll bar. -
Field Summary
Fields declared in class javafx.scene.layout.Region
USE_COMPUTED_SIZE, USE_PREF_SIZE
Fields declared in class javafx.scene.Node
BASELINE_OFFSET_SAME_AS_HEIGHT
-
Constructor Summary
ConstructorsConstructorDescriptionCreates a new ScrollPane.ScrollPane
(Node content) Creates a new ScrollPane. -
Method Summary
Modifier and TypeMethodDescriptionfinal ObjectProperty
<Node> The node used as the content of this ScrollPane.final BooleanProperty
If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport.final BooleanProperty
If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport.static List
<CssMetaData<? extends Styleable, ?>> Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.final Node
Gets the value of thecontent
property.List
<CssMetaData<? extends Styleable, ?>> Gets the unmodifiable list of the control's CSS-styleable properties.Gets the value of thehbarPolicy
property.final double
getHmax()
Gets the value of thehmax
property.final double
getHmin()
Gets the value of thehmin
property.final double
Gets the value of thehvalue
property.protected Boolean
Returns the initial focus traversable state of this control, for use by the JavaFX CSS engine to correctly set its initial value.final double
Gets the value of theminViewportHeight
property.final double
Gets the value of theminViewportWidth
property.final double
Gets the value of theprefViewportHeight
property.final double
Gets the value of theprefViewportWidth
property.Gets the value of thevbarPolicy
property.final Bounds
Gets the value of theviewportBounds
property.final double
getVmax()
Gets the value of thevmax
property.final double
getVmin()
Gets the value of thevmin
property.final double
Gets the value of thevvalue
property.Specifies the policy for showing the horizontal scroll bar.final DoubleProperty
The maximum allowablehvalue
for this ScrollPane.final DoubleProperty
The minimum allowablehvalue
for this ScrollPane.final DoubleProperty
The current horizontal scroll position of the ScrollPane.final boolean
Gets the value of thefitToHeight
property.final boolean
Gets the value of thefitToWidth
property.final boolean
Gets the value of thepannable
property.final DoubleProperty
Specify the minimum height of the ScrollPane Viewport.final DoubleProperty
Specify the minimum width of the ScrollPane Viewport.final BooleanProperty
Specifies whether the user should be able to pan the viewport by using the mouse.final DoubleProperty
Specify the preferred height of the ScrollPane Viewport.final DoubleProperty
Specify the preferred width of the ScrollPane Viewport.final void
setContent
(Node value) Sets the value of thecontent
property.final void
setFitToHeight
(boolean value) Sets the value of thefitToHeight
property.final void
setFitToWidth
(boolean value) Sets the value of thefitToWidth
property.final void
Sets the value of thehbarPolicy
property.final void
setHmax
(double value) Sets the value of thehmax
property.final void
setHmin
(double value) Sets the value of thehmin
property.final void
setHvalue
(double value) Sets the value of thehvalue
property.final void
setMinViewportHeight
(double value) Sets the value of theminViewportHeight
property.final void
setMinViewportWidth
(double value) Sets the value of theminViewportWidth
property.final void
setPannable
(boolean value) Sets the value of thepannable
property.final void
setPrefViewportHeight
(double value) Sets the value of theprefViewportHeight
property.final void
setPrefViewportWidth
(double value) Sets the value of theprefViewportWidth
property.final void
Sets the value of thevbarPolicy
property.final void
setViewportBounds
(Bounds value) Sets the value of theviewportBounds
property.final void
setVmax
(double value) Sets the value of thevmax
property.final void
setVmin
(double value) Sets the value of thevmin
property.final void
setVvalue
(double value) Sets the value of thevvalue
property.Specifies the policy for showing the vertical scroll bar.final ObjectProperty
<Bounds> The actual Bounds of the ScrollPane Viewport.final DoubleProperty
The maximum allowablevvalue
for this ScrollPane.final DoubleProperty
The minimum allowablevvalue
for this ScrollPane.final DoubleProperty
The current vertical scroll position of the ScrollPane.Methods declared in class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, contextMenuProperty, createDefaultSkin, getContextMenu, getCssMetaData, getSkin, getTooltip, isResizable, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods declared in class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, computePrefHeight, computePrefWidth, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods declared in class javafx.scene.Parent
getBaselineOffset, getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, layoutChildren, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods declared in class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, executeAccessibleAction, fireEvent, focusedProperty, focusTraversableProperty, focusVisibleProperty, focusWithinProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isFocusVisible, isFocusWithin, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookup, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, queryAccessibleAttribute, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
Methods declared in class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods declared in interface javafx.css.Styleable
getStyleableNode
-
Property Details
-
hbarPolicy
Specifies the policy for showing the horizontal scroll bar.- See Also:
-
vbarPolicy
Specifies the policy for showing the vertical scroll bar.- See Also:
-
content
The node used as the content of this ScrollPane.- See Also:
-
hvalue
The current horizontal scroll position of the ScrollPane. This value may be set by the application to scroll the view programatically. The ScrollPane will update this value whenever the viewport is scrolled or panned by the user. This value must always be within the range ofhmin
tohmax
. Whenhvalue
equalshmin
, the contained node is positioned so that its layoutBoundsminX
is visible. Whenhvalue
equalshmax
, the contained node is positioned so that its layoutBoundsmaxX
is visible. Whenhvalue
is betweenhmin
andhmax
, the contained node is positioned proportionally between layoutBoundsminX
and layoutBoundsmaxX
.- See Also:
-
vvalue
The current vertical scroll position of the ScrollPane. This value may be set by the application to scroll the view programatically. The ScrollPane will update this value whenever the viewport is scrolled or panned by the user. This value must always be within the range ofvmin
tovmax
. Whenvvalue
equalsvmin
, the contained node is positioned so that its layoutBoundsminY
is visible. Whenvvalue
equalsvmax
, the contained node is positioned so that its layoutBoundsmaxY
is visible. Whenvvalue
is betweenvmin
andvmax
, the contained node is positioned proportionally between layoutBoundsminY
and layoutBoundsmaxY
.- See Also:
-
hmin
The minimum allowablehvalue
for this ScrollPane. Default value is 0.- See Also:
-
vmin
The minimum allowablevvalue
for this ScrollPane. Default value is 0.- See Also:
-
hmax
The maximum allowablehvalue
for this ScrollPane. Default value is 1.- See Also:
-
vmax
The maximum allowablevvalue
for this ScrollPane. Default value is 1.- See Also:
-
fitToWidth
If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.- See Also:
-
fitToHeight
If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.- See Also:
-
pannable
Specifies whether the user should be able to pan the viewport by using the mouse. If mouse events reach the ScrollPane (that is, if mouse events are not blocked by the contained node or one of its children) thenpannable
is consulted to determine if the events should be used for panning.- See Also:
-
prefViewportWidth
Specify the preferred width of the ScrollPane Viewport. This is the width that will be available to the content node. The overall width of the ScrollPane is the ViewportWidth + padding- See Also:
-
prefViewportHeight
Specify the preferred height of the ScrollPane Viewport. This is the height that will be available to the content node. The overall height of the ScrollPane is the ViewportHeight + padding- See Also:
-
minViewportWidth
Specify the minimum width of the ScrollPane Viewport. This is the width that will be available to the content node.- Since:
- JavaFX 8u40
- See Also:
-
minViewportHeight
Specify the minimum height of the ScrollPane Viewport. This is the height that will be available to the content node.- Since:
- JavaFX 8u40
- See Also:
-
viewportBounds
The actual Bounds of the ScrollPane Viewport. This is the Bounds of the content node.- See Also:
-
-
Constructor Details
-
ScrollPane
public ScrollPane()Creates a new ScrollPane. -
ScrollPane
Creates a new ScrollPane.- Parameters:
content
- the initial content for the ScrollPane- Since:
- JavaFX 8.0
-
-
Method Details
-
setHbarPolicy
Sets the value of thehbarPolicy
property.- Property description:
- Specifies the policy for showing the horizontal scroll bar.
- Parameters:
value
- the value for thehbarPolicy
property- See Also:
-
getHbarPolicy
Gets the value of thehbarPolicy
property.- Property description:
- Specifies the policy for showing the horizontal scroll bar.
- Returns:
- the value of the
hbarPolicy
property - See Also:
-
hbarPolicyProperty
Specifies the policy for showing the horizontal scroll bar.- Returns:
- the
hbarPolicy
property - See Also:
-
setVbarPolicy
Sets the value of thevbarPolicy
property.- Property description:
- Specifies the policy for showing the vertical scroll bar.
- Parameters:
value
- the value for thevbarPolicy
property- See Also:
-
getVbarPolicy
Gets the value of thevbarPolicy
property.- Property description:
- Specifies the policy for showing the vertical scroll bar.
- Returns:
- the value of the
vbarPolicy
property - See Also:
-
vbarPolicyProperty
Specifies the policy for showing the vertical scroll bar.- Returns:
- the
vbarPolicy
property - See Also:
-
setContent
Sets the value of thecontent
property.- Property description:
- The node used as the content of this ScrollPane.
- Parameters:
value
- the value for thecontent
property- See Also:
-
getContent
Gets the value of thecontent
property.- Property description:
- The node used as the content of this ScrollPane.
- Returns:
- the value of the
content
property - See Also:
-
contentProperty
The node used as the content of this ScrollPane.- Returns:
- the
content
property - See Also:
-
setHvalue
public final void setHvalue(double value) Sets the value of thehvalue
property.- Property description:
- The current horizontal scroll position of the ScrollPane. This value
may be set by the application to scroll the view programatically.
The ScrollPane will update this value whenever the viewport is
scrolled or panned by the user. This value must always be within
the range of
hmin
tohmax
. Whenhvalue
equalshmin
, the contained node is positioned so that its layoutBoundsminX
is visible. Whenhvalue
equalshmax
, the contained node is positioned so that its layoutBoundsmaxX
is visible. Whenhvalue
is betweenhmin
andhmax
, the contained node is positioned proportionally between layoutBoundsminX
and layoutBoundsmaxX
. - Parameters:
value
- the value for thehvalue
property- See Also:
-
getHvalue
public final double getHvalue()Gets the value of thehvalue
property.- Property description:
- The current horizontal scroll position of the ScrollPane. This value
may be set by the application to scroll the view programatically.
The ScrollPane will update this value whenever the viewport is
scrolled or panned by the user. This value must always be within
the range of
hmin
tohmax
. Whenhvalue
equalshmin
, the contained node is positioned so that its layoutBoundsminX
is visible. Whenhvalue
equalshmax
, the contained node is positioned so that its layoutBoundsmaxX
is visible. Whenhvalue
is betweenhmin
andhmax
, the contained node is positioned proportionally between layoutBoundsminX
and layoutBoundsmaxX
. - Returns:
- the value of the
hvalue
property - See Also:
-
hvalueProperty
The current horizontal scroll position of the ScrollPane. This value may be set by the application to scroll the view programatically. The ScrollPane will update this value whenever the viewport is scrolled or panned by the user. This value must always be within the range ofhmin
tohmax
. Whenhvalue
equalshmin
, the contained node is positioned so that its layoutBoundsminX
is visible. Whenhvalue
equalshmax
, the contained node is positioned so that its layoutBoundsmaxX
is visible. Whenhvalue
is betweenhmin
andhmax
, the contained node is positioned proportionally between layoutBoundsminX
and layoutBoundsmaxX
.- Returns:
- the
hvalue
property - See Also:
-
setVvalue
public final void setVvalue(double value) Sets the value of thevvalue
property.- Property description:
- The current vertical scroll position of the ScrollPane. This value
may be set by the application to scroll the view programatically.
The ScrollPane will update this value whenever the viewport is
scrolled or panned by the user. This value must always be within
the range of
vmin
tovmax
. Whenvvalue
equalsvmin
, the contained node is positioned so that its layoutBoundsminY
is visible. Whenvvalue
equalsvmax
, the contained node is positioned so that its layoutBoundsmaxY
is visible. Whenvvalue
is betweenvmin
andvmax
, the contained node is positioned proportionally between layoutBoundsminY
and layoutBoundsmaxY
. - Parameters:
value
- the value for thevvalue
property- See Also:
-
getVvalue
public final double getVvalue()Gets the value of thevvalue
property.- Property description:
- The current vertical scroll position of the ScrollPane. This value
may be set by the application to scroll the view programatically.
The ScrollPane will update this value whenever the viewport is
scrolled or panned by the user. This value must always be within
the range of
vmin
tovmax
. Whenvvalue
equalsvmin
, the contained node is positioned so that its layoutBoundsminY
is visible. Whenvvalue
equalsvmax
, the contained node is positioned so that its layoutBoundsmaxY
is visible. Whenvvalue
is betweenvmin
andvmax
, the contained node is positioned proportionally between layoutBoundsminY
and layoutBoundsmaxY
. - Returns:
- the value of the
vvalue
property - See Also:
-
vvalueProperty
The current vertical scroll position of the ScrollPane. This value may be set by the application to scroll the view programatically. The ScrollPane will update this value whenever the viewport is scrolled or panned by the user. This value must always be within the range ofvmin
tovmax
. Whenvvalue
equalsvmin
, the contained node is positioned so that its layoutBoundsminY
is visible. Whenvvalue
equalsvmax
, the contained node is positioned so that its layoutBoundsmaxY
is visible. Whenvvalue
is betweenvmin
andvmax
, the contained node is positioned proportionally between layoutBoundsminY
and layoutBoundsmaxY
.- Returns:
- the
vvalue
property - See Also:
-
setHmin
public final void setHmin(double value) Sets the value of thehmin
property.- Property description:
- The minimum allowable
hvalue
for this ScrollPane. Default value is 0. - Parameters:
value
- the value for thehmin
property- See Also:
-
getHmin
public final double getHmin()Gets the value of thehmin
property.- Property description:
- The minimum allowable
hvalue
for this ScrollPane. Default value is 0. - Returns:
- the value of the
hmin
property - See Also:
-
hminProperty
The minimum allowablehvalue
for this ScrollPane. Default value is 0.- Returns:
- the
hmin
property - See Also:
-
setVmin
public final void setVmin(double value) Sets the value of thevmin
property.- Property description:
- The minimum allowable
vvalue
for this ScrollPane. Default value is 0. - Parameters:
value
- the value for thevmin
property- See Also:
-
getVmin
public final double getVmin()Gets the value of thevmin
property.- Property description:
- The minimum allowable
vvalue
for this ScrollPane. Default value is 0. - Returns:
- the value of the
vmin
property - See Also:
-
vminProperty
The minimum allowablevvalue
for this ScrollPane. Default value is 0.- Returns:
- the
vmin
property - See Also:
-
setHmax
public final void setHmax(double value) Sets the value of thehmax
property.- Property description:
- The maximum allowable
hvalue
for this ScrollPane. Default value is 1. - Parameters:
value
- the value for thehmax
property- See Also:
-
getHmax
public final double getHmax()Gets the value of thehmax
property.- Property description:
- The maximum allowable
hvalue
for this ScrollPane. Default value is 1. - Returns:
- the value of the
hmax
property - See Also:
-
hmaxProperty
The maximum allowablehvalue
for this ScrollPane. Default value is 1.- Returns:
- the
hmax
property - See Also:
-
setVmax
public final void setVmax(double value) Sets the value of thevmax
property.- Property description:
- The maximum allowable
vvalue
for this ScrollPane. Default value is 1. - Parameters:
value
- the value for thevmax
property- See Also:
-
getVmax
public final double getVmax()Gets the value of thevmax
property.- Property description:
- The maximum allowable
vvalue
for this ScrollPane. Default value is 1. - Returns:
- the value of the
vmax
property - See Also:
-
vmaxProperty
The maximum allowablevvalue
for this ScrollPane. Default value is 1.- Returns:
- the
vmax
property - See Also:
-
setFitToWidth
public final void setFitToWidth(boolean value) Sets the value of thefitToWidth
property.- Property description:
- If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.
- Parameters:
value
- the value for thefitToWidth
property- See Also:
-
isFitToWidth
public final boolean isFitToWidth()Gets the value of thefitToWidth
property.- Property description:
- If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.
- Returns:
- the value of the
fitToWidth
property - See Also:
-
fitToWidthProperty
If true and if the contained node is a Resizable, then the node will be kept resized to match the width of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.- Returns:
- the
fitToWidth
property - See Also:
-
setFitToHeight
public final void setFitToHeight(boolean value) Sets the value of thefitToHeight
property.- Property description:
- If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.
- Parameters:
value
- the value for thefitToHeight
property- See Also:
-
isFitToHeight
public final boolean isFitToHeight()Gets the value of thefitToHeight
property.- Property description:
- If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.
- Returns:
- the value of the
fitToHeight
property - See Also:
-
fitToHeightProperty
If true and if the contained node is a Resizable, then the node will be kept resized to match the height of the ScrollPane's viewport. If the contained node is not a Resizable, this value is ignored.- Returns:
- the
fitToHeight
property - See Also:
-
setPannable
public final void setPannable(boolean value) Sets the value of thepannable
property.- Property description:
- Specifies whether the user should be able to pan the viewport by using
the mouse. If mouse events reach the ScrollPane (that is, if mouse
events are not blocked by the contained node or one of its children)
then
pannable
is consulted to determine if the events should be used for panning. - Parameters:
value
- the value for thepannable
property- See Also:
-
isPannable
public final boolean isPannable()Gets the value of thepannable
property.- Property description:
- Specifies whether the user should be able to pan the viewport by using
the mouse. If mouse events reach the ScrollPane (that is, if mouse
events are not blocked by the contained node or one of its children)
then
pannable
is consulted to determine if the events should be used for panning. - Returns:
- the value of the
pannable
property - See Also:
-
pannableProperty
Specifies whether the user should be able to pan the viewport by using the mouse. If mouse events reach the ScrollPane (that is, if mouse events are not blocked by the contained node or one of its children) thenpannable
is consulted to determine if the events should be used for panning.- Returns:
- the
pannable
property - See Also:
-
setPrefViewportWidth
public final void setPrefViewportWidth(double value) Sets the value of theprefViewportWidth
property.- Property description:
- Specify the preferred width of the ScrollPane Viewport. This is the width that will be available to the content node. The overall width of the ScrollPane is the ViewportWidth + padding
- Parameters:
value
- the value for theprefViewportWidth
property- See Also:
-
getPrefViewportWidth
public final double getPrefViewportWidth()Gets the value of theprefViewportWidth
property.- Property description:
- Specify the preferred width of the ScrollPane Viewport. This is the width that will be available to the content node. The overall width of the ScrollPane is the ViewportWidth + padding
- Returns:
- the value of the
prefViewportWidth
property - See Also:
-
prefViewportWidthProperty
Specify the preferred width of the ScrollPane Viewport. This is the width that will be available to the content node. The overall width of the ScrollPane is the ViewportWidth + padding- Returns:
- the
prefViewportWidth
property - See Also:
-
setPrefViewportHeight
public final void setPrefViewportHeight(double value) Sets the value of theprefViewportHeight
property.- Property description:
- Specify the preferred height of the ScrollPane Viewport. This is the height that will be available to the content node. The overall height of the ScrollPane is the ViewportHeight + padding
- Parameters:
value
- the value for theprefViewportHeight
property- See Also:
-
getPrefViewportHeight
public final double getPrefViewportHeight()Gets the value of theprefViewportHeight
property.- Property description:
- Specify the preferred height of the ScrollPane Viewport. This is the height that will be available to the content node. The overall height of the ScrollPane is the ViewportHeight + padding
- Returns:
- the value of the
prefViewportHeight
property - See Also:
-
prefViewportHeightProperty
Specify the preferred height of the ScrollPane Viewport. This is the height that will be available to the content node. The overall height of the ScrollPane is the ViewportHeight + padding- Returns:
- the
prefViewportHeight
property - See Also:
-
setMinViewportWidth
public final void setMinViewportWidth(double value) Sets the value of theminViewportWidth
property.- Property description:
- Specify the minimum width of the ScrollPane Viewport. This is the width that will be available to the content node.
- Parameters:
value
- the value for theminViewportWidth
property- Since:
- JavaFX 8u40
- See Also:
-
getMinViewportWidth
public final double getMinViewportWidth()Gets the value of theminViewportWidth
property.- Property description:
- Specify the minimum width of the ScrollPane Viewport. This is the width that will be available to the content node.
- Returns:
- the value of the
minViewportWidth
property - Since:
- JavaFX 8u40
- See Also:
-
minViewportWidthProperty
Specify the minimum width of the ScrollPane Viewport. This is the width that will be available to the content node.- Returns:
- the
minViewportWidth
property - Since:
- JavaFX 8u40
- See Also:
-
setMinViewportHeight
public final void setMinViewportHeight(double value) Sets the value of theminViewportHeight
property.- Property description:
- Specify the minimum height of the ScrollPane Viewport. This is the height that will be available to the content node.
- Parameters:
value
- the value for theminViewportHeight
property- Since:
- JavaFX 8u40
- See Also:
-
getMinViewportHeight
public final double getMinViewportHeight()Gets the value of theminViewportHeight
property.- Property description:
- Specify the minimum height of the ScrollPane Viewport. This is the height that will be available to the content node.
- Returns:
- the value of the
minViewportHeight
property - Since:
- JavaFX 8u40
- See Also:
-
minViewportHeightProperty
Specify the minimum height of the ScrollPane Viewport. This is the height that will be available to the content node.- Returns:
- the
minViewportHeight
property - Since:
- JavaFX 8u40
- See Also:
-
setViewportBounds
Sets the value of theviewportBounds
property.- Property description:
- The actual Bounds of the ScrollPane Viewport. This is the Bounds of the content node.
- Parameters:
value
- the value for theviewportBounds
property- See Also:
-
getViewportBounds
Gets the value of theviewportBounds
property.- Property description:
- The actual Bounds of the ScrollPane Viewport. This is the Bounds of the content node.
- Returns:
- the value of the
viewportBounds
property - See Also:
-
viewportBoundsProperty
The actual Bounds of the ScrollPane Viewport. This is the Bounds of the content node.- Returns:
- the
viewportBounds
property - See Also:
-
getClassCssMetaData
Gets theCssMetaData
associated with this class, which may include theCssMetaData
of its superclasses.- Returns:
- the
CssMetaData
- Since:
- JavaFX 8.0
-
getControlCssMetaData
Gets the unmodifiable list of the control's CSS-styleable properties.- Overrides:
getControlCssMetaData
in classControl
- Returns:
- the unmodifiable list of the control's CSS-styleable properties
- Since:
- JavaFX 8.0
-
getInitialFocusTraversable
Returns the initial focus traversable state of this control, for use by the JavaFX CSS engine to correctly set its initial value. This method is overridden as by default UI controls have focus traversable set to true, but that is not appropriate for this control.- Overrides:
getInitialFocusTraversable
in classControl
- Returns:
- the initial focus traversable state of this control
- Since:
- 9
-