Class RadioMenuItem
- All Implemented Interfaces:
Styleable
,EventTarget
,Toggle
A RadioMenuItem is a MenuItem
that can be toggled (it uses
the Toggle
mixin). This means that
RadioMenuItem has an API very similar in nature to other controls that use
Toggle
, such as
RadioButton
and
ToggleButton
. RadioMenuItem is
specifically designed for use within a Menu
, so refer to that class
API documentation for more information on how to add a RadioMenuItem into it.
To create a simple, ungrouped RadioMenuItem, do the following:
RadioMenuItem radioItem = new RadioMenuItem("radio text");
radioItem.setSelected(false);
radioItem.setOnAction(e -> System.out.println("radio toggled"));
The problem with the example above is that this offers no benefit over using
a normal MenuItem. As already mentioned, the purpose of a
RadioMenuItem is to offer
multiple choices to the user, and only allow for one of these choices to be
selected at any one time (i.e. the selection should be mutually exclusive).
To achieve this, you can place zero or more RadioMenuItem's into groups. When
in groups, only one RadioMenuItem at a time within that group can be selected.
To put two RadioMenuItem instances into the same group, simply assign them
both the same value for toggleGroup
. For example:
ToggleGroup toggleGroup = new ToggleGroup();
RadioMenuItem radioItem1 = new RadioMenuItem("Option 1");
radioItem1.setOnAction(e -> System.out.println("radio1 toggled"));
radioItem1.setToggleGroup(toggleGroup);
RadioMenuItem radioItem2 = new RadioMenuItem("Option 2");
radioItem2.setOnAction(e -> System.out.println("radio2 toggled"));
radioItem2.setToggleGroup(toggleGroup);
Menu menu = new Menu("Selection");
menu.getItems().addAll(radioItem1, radioItem2);
MenuBar menuBar = new MenuBar(menu);
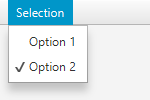
In this example, with both RadioMenuItem's assigned to the same
ToggleGroup
, only one item may be
selected at any one time, and should
the selection change, the ToggleGroup will take care of deselecting the
previous item.
- Since:
- JavaFX 2.0
- See Also:
-
Property Summary
PropertiesTypePropertyDescriptionfinal BooleanProperty
The selected state for thisToggle
.final ObjectProperty
<ToggleGroup> Represents theToggleGroup
that this RadioMenuItem belongs to.Properties declared in class javafx.scene.control.MenuItem
accelerator, disable, graphic, id, mnemonicParsing, onAction, onMenuValidation, parentMenu, parentPopup, style, text, visible
-
Field Summary
Fields declared in class javafx.scene.control.MenuItem
MENU_VALIDATION_EVENT
-
Constructor Summary
ConstructorsConstructorDescriptionConstructs a RadioMenuItem with no display text.RadioMenuItem
(String text) Constructs a RadioMenuItem and sets the display text with the specified text.RadioMenuItem
(String text, Node graphic) Constructs a RadioMenuItem and sets the display text with the specified text and sets the graphicNode
to the given node. -
Method Summary
Modifier and TypeMethodDescriptionfinal ToggleGroup
Gets the value of thetoggleGroup
property.final boolean
Gets the value of theselected
property.final BooleanProperty
The selected state for thisToggle
.final void
setSelected
(boolean value) Sets the value of theselected
property.final void
setToggleGroup
(ToggleGroup value) Sets the value of thetoggleGroup
property.final ObjectProperty
<ToggleGroup> Represents theToggleGroup
that this RadioMenuItem belongs to.Methods declared in class javafx.scene.control.MenuItem
acceleratorProperty, buildEventDispatchChain, disableProperty, fire, getAccelerator, getCssMetaData, getGraphic, getId, getOnAction, getOnMenuValidation, getParentMenu, getParentPopup, getProperties, getPseudoClassStates, getStyle, getStyleableParent, getStyleClass, getText, getTypeSelector, getUserData, graphicProperty, idProperty, isDisable, isMnemonicParsing, isVisible, mnemonicParsingProperty, onActionProperty, onMenuValidationProperty, parentMenuProperty, parentPopupProperty, setAccelerator, setDisable, setGraphic, setId, setMnemonicParsing, setOnAction, setOnMenuValidation, setParentMenu, setParentPopup, setStyle, setText, setUserData, setVisible, styleProperty, textProperty, visibleProperty
Methods declared in class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods declared in interface javafx.event.EventTarget
addEventFilter, addEventHandler, removeEventFilter, removeEventHandler
Methods declared in interface javafx.css.Styleable
getStyleableNode
Methods declared in interface javafx.scene.control.Toggle
getProperties, getUserData, setUserData
-
Property Details
-
toggleGroup
Represents theToggleGroup
that this RadioMenuItem belongs to.- Specified by:
toggleGroupProperty
in interfaceToggle
- Returns:
- the toggle group property
- See Also:
-
selected
- Specified by:
selectedProperty
in interfaceToggle
- Returns:
- the selected property
- See Also:
-
-
Constructor Details
-
RadioMenuItem
public RadioMenuItem()Constructs a RadioMenuItem with no display text. -
RadioMenuItem
Constructs a RadioMenuItem and sets the display text with the specified text.- Parameters:
text
- the display text
-
RadioMenuItem
-
-
Method Details
-
setToggleGroup
Sets the value of thetoggleGroup
property.- Specified by:
setToggleGroup
in interfaceToggle
- Property description:
- Represents the
ToggleGroup
that this RadioMenuItem belongs to. - Parameters:
value
- the value for thetoggleGroup
property- See Also:
-
getToggleGroup
Gets the value of thetoggleGroup
property.- Specified by:
getToggleGroup
in interfaceToggle
- Property description:
- Represents the
ToggleGroup
that this RadioMenuItem belongs to. - Returns:
- the value of the
toggleGroup
property - See Also:
-
toggleGroupProperty
Represents theToggleGroup
that this RadioMenuItem belongs to.- Specified by:
toggleGroupProperty
in interfaceToggle
- Returns:
- the
toggleGroup
property - See Also:
-
setSelected
public final void setSelected(boolean value) Sets the value of theselected
property.- Specified by:
setSelected
in interfaceToggle
- Property description:
- Parameters:
value
- the value for theselected
property- See Also:
-
isSelected
public final boolean isSelected()Gets the value of theselected
property.- Specified by:
isSelected
in interfaceToggle
- Property description:
- Returns:
- the value of the
selected
property - See Also:
-
selectedProperty
Description copied from interface:Toggle
The selected state for thisToggle
.- Specified by:
selectedProperty
in interfaceToggle
- Returns:
- the
selected
property - See Also:
-