- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
A popup control containing an ObservableList of menu items. The items
ObservableList allows for any MenuItem
type to be inserted,
including its subclasses Menu
, MenuItem
, RadioMenuItem
, CheckMenuItem
and
CustomMenuItem
. If an arbitrary Node needs to be
inserted into a menu, a CustomMenuItem can be used. One exception to this general rule is that
SeparatorMenuItem
could be used for inserting a separator.
A common use case for this class is creating and showing context menus to users. To create a context menu using ContextMenu you can do the following:
final ContextMenu contextMenu = new ContextMenu();
contextMenu.setOnShowing(new EventHandler<WindowEvent>() {
public void handle(WindowEvent e) {
System.out.println("showing");
}
});
contextMenu.setOnShown(new EventHandler<WindowEvent>() {
public void handle(WindowEvent e) {
System.out.println("shown");
}
});
MenuItem item1 = new MenuItem("About");
item1.setOnAction(new EventHandler<ActionEvent>() {
public void handle(ActionEvent e) {
System.out.println("About");
}
});
MenuItem item2 = new MenuItem("Preferences");
item2.setOnAction(new EventHandler<ActionEvent>() {
public void handle(ActionEvent e) {
System.out.println("Preferences");
}
});
contextMenu.getItems().addAll(item1, item2);
final TextField textField = new TextField("Type Something");
textField.setContextMenu(contextMenu);
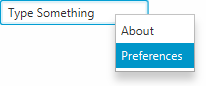
Control.setContextMenu(javafx.scene.control.ContextMenu)
convenience
method can be used to set a context menu on on any control. The example above results in the
context menu being displayed on the right Side
of the TextField. Alternatively, an event handler can also be set on the control
to invoke the context menu as shown below.
textField.setOnAction(new EventHandler<ActionEvent>() {
public void handle(ActionEvent e) {
contextMenu.show(textField, Side.BOTTOM, 0, 0);
}
});
Group root = (Group) scene.getRoot();
root.getChildren().add(textField);
In this example, the context menu is shown when the user clicks on the
Button
(of course, you should use the
MenuButton
control to do this rather than doing the above).
Note that the show function used in the code sample
above will result in the ContextMenu appearing directly beneath the
TextField. You can vary the Side
to get the results you expect.
- Since:
- JavaFX 2.0
- See Also:
-
Nested Class Summary
Nested classes/interfaces declared in class javafx.scene.control.PopupControl
PopupControl.CSSBridge
Nested classes/interfaces declared in class javafx.stage.PopupWindow
PopupWindow.AnchorLocation
-
Property Summary
PropertiesTypePropertyDescriptionfinal ObjectProperty
<EventHandler<ActionEvent>> Callback function to be informed when an item contained within thisContextMenu
has been activated.Properties declared in class javafx.scene.control.PopupControl
id, maxHeight, maxWidth, minHeight, minWidth, prefHeight, prefWidth, skin, style
Properties declared in class javafx.stage.PopupWindow
anchorLocation, anchorX, anchorY, autoFix, autoHide, consumeAutoHidingEvents, hideOnEscape, onAutoHide, ownerNode, ownerWindow
Properties declared in class javafx.stage.Window
eventDispatcher, focused, forceIntegerRenderScale, height, onCloseRequest, onHidden, onHiding, onShowing, onShown, opacity, outputScaleX, outputScaleY, renderScaleX, renderScaleY, scene, showing, width, x, y
-
Field Summary
Fields declared in class javafx.scene.control.PopupControl
bridge, USE_COMPUTED_SIZE, USE_PREF_SIZE
-
Constructor Summary
ConstructorsConstructorDescriptionCreate a new ContextMenuContextMenu
(MenuItem... items) Create a new ContextMenu initialized with the given items -
Method Summary
Modifier and TypeMethodDescriptionfinal ObservableList
<MenuItem> getItems()
The menu items on the context menu.final EventHandler
<ActionEvent> Gets the value of theonAction
property.void
hide()
Hides thisContextMenu
and any visible submenus, assuming that when this function is called that theContextMenu
was showing.final ObjectProperty
<EventHandler<ActionEvent>> Callback function to be informed when an item contained within thisContextMenu
has been activated.final void
setOnAction
(EventHandler<ActionEvent> value) Sets the value of theonAction
property.void
Shows theContextMenu
at the specified screen coordinates.void
Shows theContextMenu
relative to the given anchor node, on the side specified by theside
parameter, and offset by the givendx
anddy
values for the x-axis and y-axis, respectively.Methods declared in class javafx.scene.control.PopupControl
createDefaultSkin, getClassCssMetaData, getCssMetaData, getId, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getPrefHeight, getPrefWidth, getPseudoClassStates, getSkin, getStyle, getStyleableParent, getStyleClass, getTypeSelector, idProperty, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, pseudoClassStateChanged, setId, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setPrefHeight, setPrefSize, setPrefWidth, setSkin, setStyle, skinProperty, styleProperty
Methods declared in class javafx.stage.PopupWindow
anchorLocationProperty, anchorXProperty, anchorYProperty, autoFixProperty, autoHideProperty, consumeAutoHidingEventsProperty, getAnchorLocation, getAnchorX, getAnchorY, getConsumeAutoHidingEvents, getOnAutoHide, getOwnerNode, getOwnerWindow, hideOnEscapeProperty, isAutoFix, isAutoHide, isHideOnEscape, onAutoHideProperty, ownerNodeProperty, ownerWindowProperty, setAnchorLocation, setAnchorX, setAnchorY, setAutoFix, setAutoHide, setConsumeAutoHidingEvents, setHideOnEscape, setOnAutoHide, setScene, show, show
Methods declared in class javafx.stage.Window
addEventFilter, addEventHandler, buildEventDispatchChain, centerOnScreen, eventDispatcherProperty, fireEvent, focusedProperty, forceIntegerRenderScaleProperty, getEventDispatcher, getHeight, getOnCloseRequest, getOnHidden, getOnHiding, getOnShowing, getOnShown, getOpacity, getOutputScaleX, getOutputScaleY, getProperties, getRenderScaleX, getRenderScaleY, getScene, getUserData, getWidth, getWindows, getX, getY, hasProperties, heightProperty, isFocused, isForceIntegerRenderScale, isShowing, onCloseRequestProperty, onHiddenProperty, onHidingProperty, onShowingProperty, onShownProperty, opacityProperty, outputScaleXProperty, outputScaleYProperty, removeEventFilter, removeEventHandler, renderScaleXProperty, renderScaleYProperty, requestFocus, sceneProperty, setEventDispatcher, setEventHandler, setForceIntegerRenderScale, setHeight, setOnCloseRequest, setOnHidden, setOnHiding, setOnShowing, setOnShown, setOpacity, setRenderScaleX, setRenderScaleY, setUserData, setWidth, setX, setY, show, showingProperty, sizeToScene, widthProperty, xProperty, yProperty
Methods declared in class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods declared in interface javafx.css.Styleable
getStyleableNode
-
Property Details
-
onAction
Callback function to be informed when an item contained within thisContextMenu
has been activated. The current implementation informs all parent menus as well, so that it is not necessary to listen to all sub menus for events.- See Also:
-
-
Constructor Details
-
ContextMenu
public ContextMenu()Create a new ContextMenu -
ContextMenu
Create a new ContextMenu initialized with the given items- Parameters:
items
- the list of menu items
-
-
Method Details
-
setOnAction
Sets the value of theonAction
property.- Property description:
- Callback function to be informed when an item contained within this
ContextMenu
has been activated. The current implementation informs all parent menus as well, so that it is not necessary to listen to all sub menus for events. - Parameters:
value
- the value for theonAction
property- See Also:
-
getOnAction
Gets the value of theonAction
property.- Property description:
- Callback function to be informed when an item contained within this
ContextMenu
has been activated. The current implementation informs all parent menus as well, so that it is not necessary to listen to all sub menus for events. - Returns:
- the value of the
onAction
property - See Also:
-
onActionProperty
Callback function to be informed when an item contained within thisContextMenu
has been activated. The current implementation informs all parent menus as well, so that it is not necessary to listen to all sub menus for events.- Returns:
- the
onAction
property - See Also:
-
getItems
The menu items on the context menu. If this ObservableList is modified at runtime, the ContextMenu will update as expected.- Returns:
- the menu items on this context menu
- See Also:
-
show
Shows theContextMenu
relative to the given anchor node, on the side specified by theside
parameter, and offset by the givendx
anddy
values for the x-axis and y-axis, respectively. If there is not enough room, the menu is moved to the opposite side and the offset is not applied.To clarify the purpose of the
side
parameter, consider that it is relative to the anchor node. As such, aside
ofTOP
would mean that the ContextMenu's bottom left corner is set to the top left corner of the anchor.This function is useful for finely tuning the position of a menu, relative to the parent node to ensure close alignment.
- Parameters:
anchor
- the anchor nodeside
- the sidedx
- the dx value for the x-axisdy
- the dy value for the y-axis
-
show
Shows theContextMenu
at the specified screen coordinates. If there is not enough room at the specified location to show theContextMenu
given its size requirements, the necessary adjustments are made to bring theContextMenu
back on screen. This also means that theContextMenu
will not span multiple monitors.- Overrides:
show
in classPopupWindow
- Parameters:
anchor
- the anchor nodescreenX
- the x position of the anchor in screen coordinatesscreenY
- the y position of the anchor in screen coordinates
-
hide
public void hide()Hides thisContextMenu
and any visible submenus, assuming that when this function is called that theContextMenu
was showing.If this
ContextMenu
is not showing, then nothing happens.- Overrides:
hide
in classPopupWindow
-