Class MenuButton
- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
- Direct Known Subclasses:
SplitMenuButton
ContextMenu
. A MenuButton shares a very similar API to the Menu
control, insofar that you set the items that should be shown in the
items
ObservableList, and there is a text
property to specify the
label shown within the MenuButton.
As mentioned, like the Menu API itself, you'll find an items
ObservableList
within which you can provide anything that extends from MenuItem
.
There are several useful subclasses of MenuItem
including
RadioMenuItem
, CheckMenuItem
, Menu
,
SeparatorMenuItem
and CustomMenuItem
.
A MenuButton can be set to show its menu on any side of the button. This is
specified using the popupSide
property. By default
the menu appears below the button. However, regardless of the popupSide specified,
if there is not enough room, the ContextMenu
will be
smartly repositioned, most probably to be on the opposite side of the
MenuButton.
Example:
MenuButton m = new MenuButton("Eats"); m.getItems().addAll(new MenuItem("Burger"), new MenuItem("Hot Dog"));
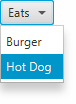
MnemonicParsing is enabled by default for MenuButton.
- Since:
- JavaFX 2.0
- See Also:
-
Property Summary
PropertiesTypePropertyDescriptionfinal ObjectProperty
<EventHandler<Event>> Called just after theContextMenu
has been hidden.final ObjectProperty
<EventHandler<Event>> Called just prior to theContextMenu
being hidden.final ObjectProperty
<EventHandler<Event>> Called just prior to theContextMenu
being shown.final ObjectProperty
<EventHandler<Event>> Called just after theContextMenu
is shown.final ObjectProperty
<Side> Indicates on which side theContextMenu
should open in relation to the MenuButton.final ReadOnlyBooleanProperty
Indicates whether theContextMenu
is currently visible.Properties declared in class javafx.scene.control.ButtonBase
armed, onAction
Properties declared in class javafx.scene.control.Labeled
alignment, contentDisplay, ellipsisString, font, graphic, graphicTextGap, labelPadding, lineSpacing, mnemonicParsing, textAlignment, textFill, textOverrun, text, textTruncated, underline, wrapText
Properties declared in class javafx.scene.control.Control
contextMenu, skin, tooltip
Properties declared in class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties declared in class javafx.scene.Parent
needsLayout
Properties declared in class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, focusVisible, focusWithin, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Field Summary
FieldsModifier and TypeFieldDescriptionCalled when the MenuButton popup has been hidden.Called when the MenuButton popup will be hidden.Called prior to the MenuButton showing its popup after the user has clicked or otherwise interacted with the MenuButton.Called after the MenuButton has shown its popup.Fields declared in class javafx.scene.layout.Region
USE_COMPUTED_SIZE, USE_PREF_SIZE
Fields declared in class javafx.scene.Node
BASELINE_OFFSET_SAME_AS_HEIGHT
-
Constructor Summary
ConstructorsConstructorDescriptionCreates a new empty menu button.MenuButton
(String text) Creates a new empty menu button with the given text to display on the button.MenuButton
(String text, Node graphic) Creates a new empty menu button with the given text and graphic to display on the button.MenuButton
(String text, Node graphic, MenuItem... items) Creates a new menu button with the given text and graphic to display on the button, and inserts the given items into theitems
list. -
Method Summary
Modifier and TypeMethodDescriptionvoid
fire()
This has no impact.final ObservableList
<MenuItem> getItems()
The items to show within this buttons menu.final EventHandler
<Event> Gets the value of theonHidden
property.final EventHandler
<Event> Gets the value of theonHiding
property.final EventHandler
<Event> Gets the value of theonShowing
property.final EventHandler
<Event> Gets the value of theonShown
property.final Side
Gets the value of thepopupSide
property.void
hide()
Hides theContextMenu
.final boolean
Gets the value of theshowing
property.final ObjectProperty
<EventHandler<Event>> Called just after theContextMenu
has been hidden.final ObjectProperty
<EventHandler<Event>> Called just prior to theContextMenu
being hidden.final ObjectProperty
<EventHandler<Event>> Called just prior to theContextMenu
being shown.final ObjectProperty
<EventHandler<Event>> Called just after theContextMenu
is shown.final ObjectProperty
<Side> Indicates on which side theContextMenu
should open in relation to the MenuButton.final void
setOnHidden
(EventHandler<Event> value) Sets the value of theonHidden
property.final void
setOnHiding
(EventHandler<Event> value) Sets the value of theonHiding
property.final void
setOnShowing
(EventHandler<Event> value) Sets the value of theonShowing
property.final void
setOnShown
(EventHandler<Event> value) Sets the value of theonShown
property.final void
setPopupSide
(Side value) Sets the value of thepopupSide
property.void
show()
Shows theContextMenu
, assuming this MenuButton is not disabled.final ReadOnlyBooleanProperty
Indicates whether theContextMenu
is currently visible.Methods declared in class javafx.scene.control.ButtonBase
arm, armedProperty, disarm, getOnAction, isArmed, onActionProperty, setOnAction
Methods declared in class javafx.scene.control.Labeled
alignmentProperty, contentDisplayProperty, ellipsisStringProperty, fontProperty, getAlignment, getClassCssMetaData, getContentBias, getContentDisplay, getControlCssMetaData, getEllipsisString, getFont, getGraphic, getGraphicTextGap, getInitialAlignment, getLabelPadding, getLineSpacing, getText, getTextAlignment, getTextFill, getTextOverrun, graphicProperty, graphicTextGapProperty, isMnemonicParsing, isTextTruncated, isUnderline, isWrapText, labelPaddingProperty, lineSpacingProperty, mnemonicParsingProperty, setAlignment, setContentDisplay, setEllipsisString, setFont, setGraphic, setGraphicTextGap, setLineSpacing, setMnemonicParsing, setText, setTextAlignment, setTextFill, setTextOverrun, setUnderline, setWrapText, textAlignmentProperty, textFillProperty, textOverrunProperty, textProperty, textTruncatedProperty, underlineProperty, wrapTextProperty
Methods declared in class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, contextMenuProperty, createDefaultSkin, getContextMenu, getCssMetaData, getInitialFocusTraversable, getSkin, getTooltip, isResizable, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods declared in class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, computePrefHeight, computePrefWidth, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getUserAgentStylesheet, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods declared in class javafx.scene.Parent
getBaselineOffset, getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, layoutChildren, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods declared in class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, executeAccessibleAction, fireEvent, focusedProperty, focusTraversableProperty, focusVisibleProperty, focusWithinProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isFocusVisible, isFocusWithin, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookup, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, queryAccessibleAttribute, relocate, removeEventFilter, removeEventHandler, requestFocus, requestFocusTraversal, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
Methods declared in class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods declared in interface javafx.css.Styleable
getStyleableNode
-
Property Details
-
showing
Indicates whether theContextMenu
is currently visible.- See Also:
-
popupSide
Indicates on which side theContextMenu
should open in relation to the MenuButton. Menu items are generally laid out vertically in either case. For example, if the menu button were in a vertical toolbar on the left edge of the application, you might changepopupSide
toSide.RIGHT
so that the popup will appear to the right of the MenuButton.- Default value:
Side.BOTTOM
- See Also:
-
onShowing
Called just prior to theContextMenu
being shown.- Since:
- 10
- See Also:
-
onShown
Called just after theContextMenu
is shown.- Since:
- 10
- See Also:
-
onHiding
Called just prior to theContextMenu
being hidden.- Since:
- 10
- See Also:
-
onHidden
Called just after theContextMenu
has been hidden.- Since:
- 10
- See Also:
-
-
Field Details
-
ON_SHOWING
-
ON_SHOWN
-
ON_HIDING
-
ON_HIDDEN
-
-
Constructor Details
-
MenuButton
public MenuButton()Creates a new empty menu button. UseLabeled.setText(String)
,Labeled.setGraphic(Node)
andgetItems()
to set the content. -
MenuButton
Creates a new empty menu button with the given text to display on the button. UseLabeled.setGraphic(Node)
andgetItems()
to set the content.- Parameters:
text
- the text to display on the menu button
-
MenuButton
Creates a new empty menu button with the given text and graphic to display on the button. UsegetItems()
to set the content.- Parameters:
text
- the text to display on the menu buttongraphic
- the graphic to display on the menu button
-
MenuButton
Creates a new menu button with the given text and graphic to display on the button, and inserts the given items into theitems
list.- Parameters:
text
- the text to display on the menu buttongraphic
- the graphic to display on the menu buttonitems
- The items to display in the popup menu.- Since:
- JavaFX 8u40
-
-
Method Details
-
getItems
The items to show within this buttons menu. If this ObservableList is modified at runtime, the Menu will update as expected.Commonly used controls include including
MenuItem
,CheckMenuItem
,RadioMenuItem
, and of courseMenu
, which if added to a menu, will become a sub menu.SeparatorMenuItem
is another commonly used Node in the Menu's items ObservableList.- Returns:
- the list of menu items within this buttons menu
-
isShowing
public final boolean isShowing()Gets the value of theshowing
property.- Property description:
- Indicates whether the
ContextMenu
is currently visible. - Returns:
- the value of the
showing
property - See Also:
-
showingProperty
Indicates whether theContextMenu
is currently visible.- Returns:
- the
showing
property - See Also:
-
setPopupSide
Sets the value of thepopupSide
property.- Property description:
- Indicates on which side the
ContextMenu
should open in relation to the MenuButton. Menu items are generally laid out vertically in either case. For example, if the menu button were in a vertical toolbar on the left edge of the application, you might changepopupSide
toSide.RIGHT
so that the popup will appear to the right of the MenuButton. - Default value:
Side.BOTTOM
- Parameters:
value
- the value for thepopupSide
property- See Also:
-
getPopupSide
Gets the value of thepopupSide
property.- Property description:
- Indicates on which side the
ContextMenu
should open in relation to the MenuButton. Menu items are generally laid out vertically in either case. For example, if the menu button were in a vertical toolbar on the left edge of the application, you might changepopupSide
toSide.RIGHT
so that the popup will appear to the right of the MenuButton. - Default value:
Side.BOTTOM
- Returns:
- the value of the
popupSide
property - See Also:
-
popupSideProperty
Indicates on which side theContextMenu
should open in relation to the MenuButton. Menu items are generally laid out vertically in either case. For example, if the menu button were in a vertical toolbar on the left edge of the application, you might changepopupSide
toSide.RIGHT
so that the popup will appear to the right of the MenuButton.- Default value:
Side.BOTTOM
- Returns:
- the
popupSide
property - See Also:
-
onShowingProperty
Called just prior to theContextMenu
being shown.- Returns:
- the on showing property
- Since:
- 10
- See Also:
-
setOnShowing
Sets the value of theonShowing
property.- Property description:
- Called just prior to the
ContextMenu
being shown. - Parameters:
value
- the value for theonShowing
property- Since:
- 10
- See Also:
-
getOnShowing
Gets the value of theonShowing
property.- Property description:
- Called just prior to the
ContextMenu
being shown. - Returns:
- the value of the
onShowing
property - Since:
- 10
- See Also:
-
onShownProperty
Called just after theContextMenu
is shown.- Returns:
- the on shown property
- Since:
- 10
- See Also:
-
setOnShown
Sets the value of theonShown
property.- Property description:
- Called just after the
ContextMenu
is shown. - Parameters:
value
- the value for theonShown
property- Since:
- 10
- See Also:
-
getOnShown
Gets the value of theonShown
property.- Property description:
- Called just after the
ContextMenu
is shown. - Returns:
- the value of the
onShown
property - Since:
- 10
- See Also:
-
onHidingProperty
Called just prior to theContextMenu
being hidden.- Returns:
- the on hiding property
- Since:
- 10
- See Also:
-
setOnHiding
Sets the value of theonHiding
property.- Property description:
- Called just prior to the
ContextMenu
being hidden. - Parameters:
value
- the value for theonHiding
property- Since:
- 10
- See Also:
-
getOnHiding
Gets the value of theonHiding
property.- Property description:
- Called just prior to the
ContextMenu
being hidden. - Returns:
- the value of the
onHiding
property - Since:
- 10
- See Also:
-
onHiddenProperty
Called just after theContextMenu
has been hidden.- Returns:
- the on hidden property
- Since:
- 10
- See Also:
-
setOnHidden
Sets the value of theonHidden
property.- Property description:
- Called just after the
ContextMenu
has been hidden. - Parameters:
value
- the value for theonHidden
property- Since:
- 10
- See Also:
-
getOnHidden
Gets the value of theonHidden
property.- Property description:
- Called just after the
ContextMenu
has been hidden. - Returns:
- the value of the
onHidden
property - Since:
- 10
- See Also:
-
show
-
hide
-
fire
-